Introducing stepzen request - Command Line GraphQL Client
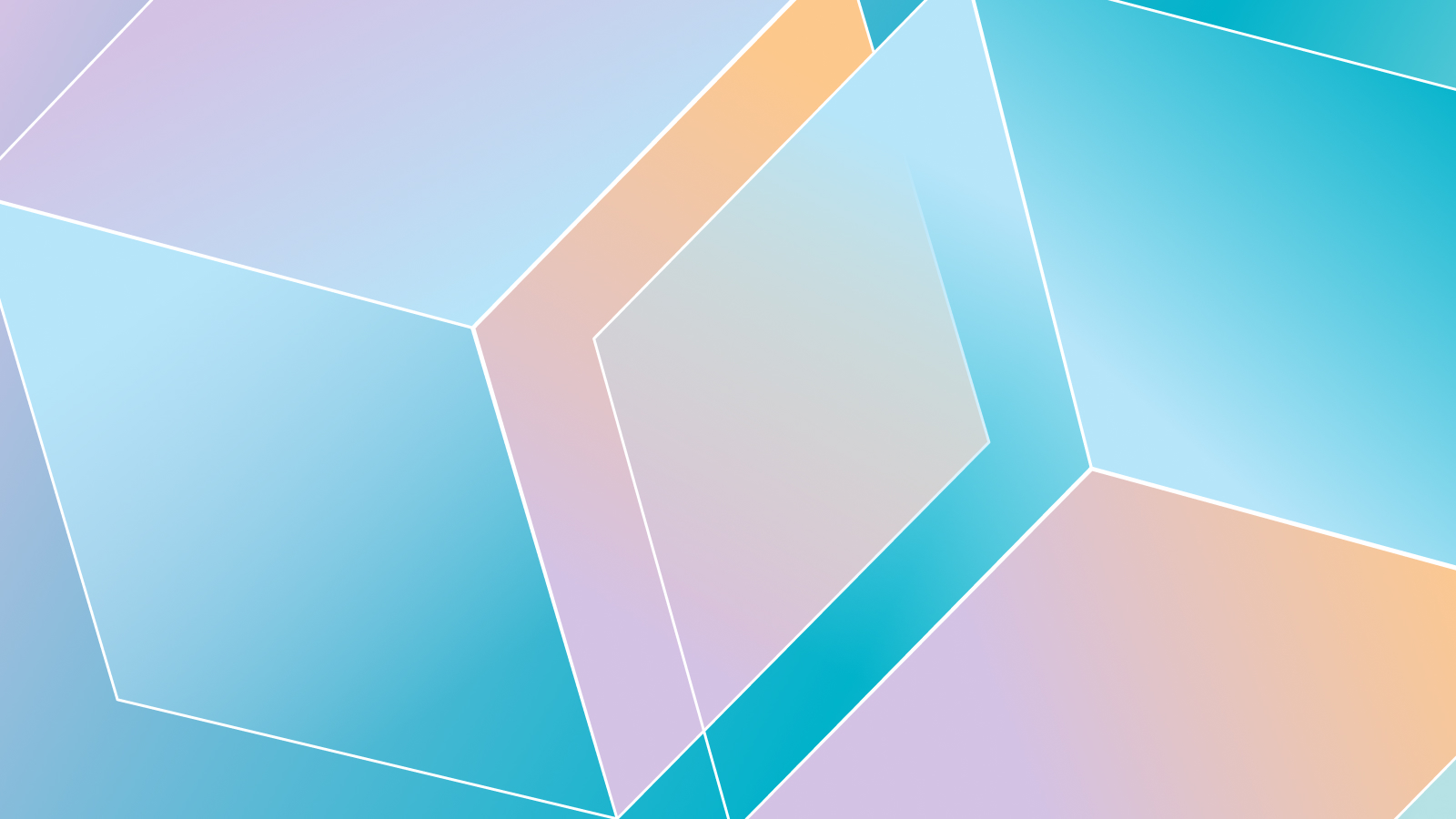
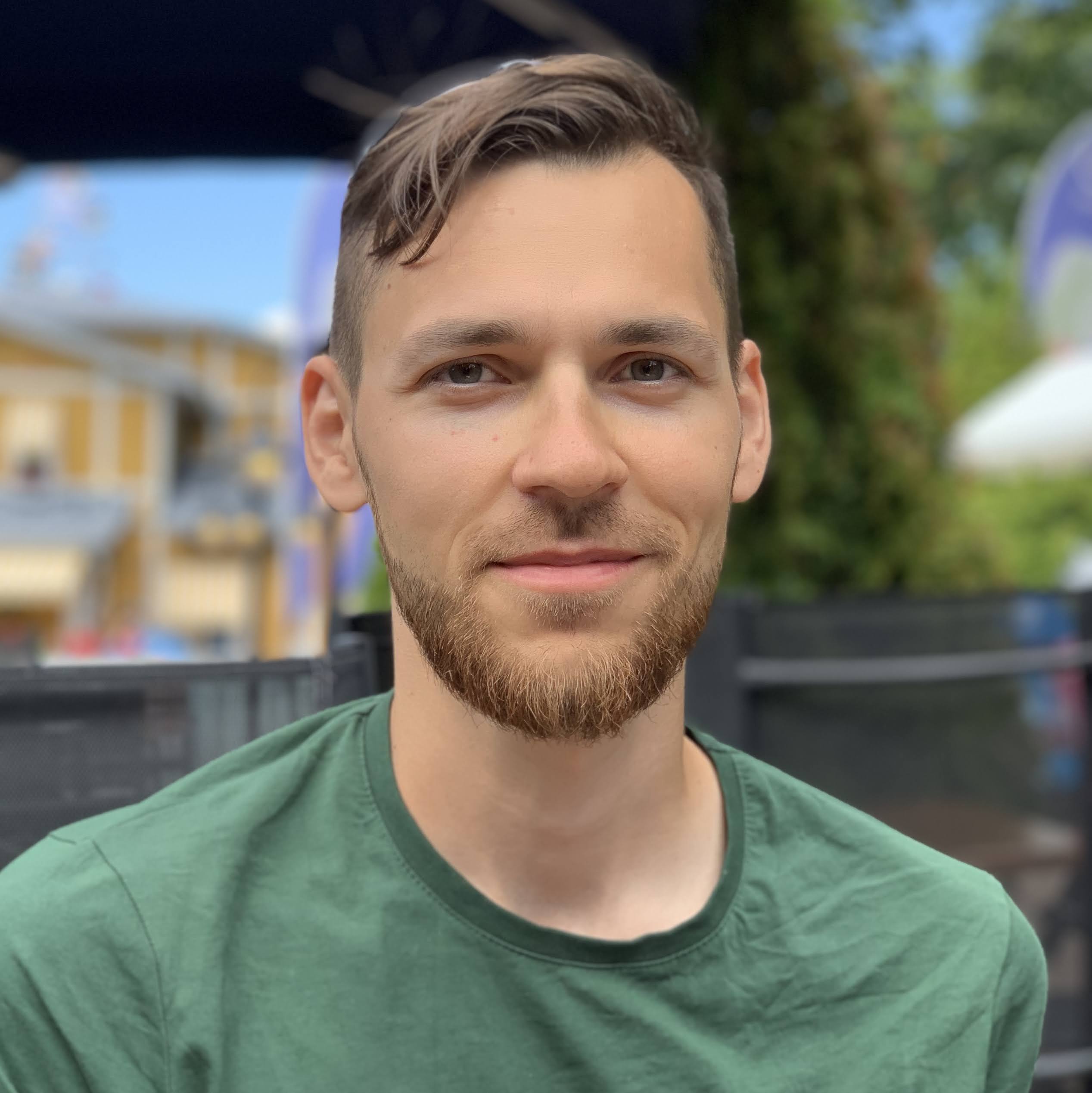
Sometimes you need to test your GraphQL API quickly, and you want to stay on your terminal to do that. A curl
command is a "swiss army knife" tool for sending network requests from the command line or terminal, and it certainly does support GraphQL. However, using curl
for this purpose is a bit verbose, and I, for one, do not always remember all the flags and headers I need to use to make it work.
With a specialized stepzen request
command, interacting with GraphQL APIs from a terminal is much easier. And, unlike curl
, stepzen request
supports GraphQL subscriptions.
Compare
curl https://braselton.stepzen.net/api/hello-world/__graphql \
--header "Authorization: Apikey $(stepzen whoami --apikey)" \
--header "Content-Type: application/json" \
--data-raw '{
"query": "query SampleQuery { __schema { description queryType { fields {name} } } }"
}'
with
stepzen request 'query SampleQuery {
__schema { description queryType { fields {name} } }
}'
Using stepzen request
In this blog, we cover how stepzen requests
works:
- Queries, Mutations and Subscriptions
- Executing Requests from a File
- Request Variables
- Customizing HTTP Headers
Queries, Mutations, and Subscriptions
When stepzen request
is used to execute a query or mutation operation, it prints the response as JSON and sets an exit code based on whether or not the response has any GraphQL errors.
For example, this is how to execute a simple mutation operation:
stepzen request 'mutation {
updateCustomerEmail(
customer_id: 5
email: "sam@example.com"
) {
email
first_name
last_name
}
}'
The output:
{
"data": {
"updateCustomerEmail": {
"email": "sam@example.com",
"first_name": "Sam",
"last_name": "Exampleson"
}
}
}
When stepzen request
is used to execute a subscription operation, it keeps running until the subscription is active and prints each event as the GraphQL server sends them.
For example, executing a subscription operation looks like this:
stepzen request 'subscription {
customer(customer_id: 5) {
email
first_name
last_name
}
}'
The output:
Subscribed to wss://braselton.stepzen.net/stepzen-subscriptions/api/hello-world/__graphql
event #1 (+549ms)
1 | {
1 | "data": {
1 | "customer": {
1 | "email": "sam.exampleson@example.com",
1 | "first_name": "Sam",
1 | "last_name": "Exampleson"
1 | }
1 | }
1 | }
event #2 (+19.9s)
2 | {
2 | "data": {
2 | "customer": {
2 | "email": "sam@example.com",
2 | "first_name": "Sam",
2 | "last_name": "Exampleson"
2 | }
2 | }
2 | }
^C
In the example above, the user terminated the subscription request by pressing Ctrl+C
on the keyboard.
Executing Requests from a File
Providing request text inline in the terminal works well for simple one-off queries, but you may find it more convenient to save them to a file for more complex or repeating requests.
With stepzen request
, you can read a request from a file using an inline shell command like cat
:
stepzen request "$(cat request.graphql)"
Which takes the operation from the file request.graphql
:
{
customer(customer_id: 5) {
email
first_name
last_name
}
}
Selecting the Operation with --operation-name
You can include multiple named operations into a single request file.
In this case, you need to provide an --operation-name
flag to the stepzen request
command to specify which operation to execute.
For example, for a request.graphql
file below:
query getUser {
customer(customer_id: 5) {
email
first_name
last_name
}
}
mutation updateUser {
updateCustomerEmail(
customer_id: 5
email: "sam@example.com"
) {
email
first_name
last_name
}
}
You can execute the getUser
query as follows:
stepzen request "$(cat request.graphql)" --operation-name getUser
Request Variables
The email and customer ID values are hard-coded straight into the request text in the examples with request files above.
In practice, you should make these values variable so that you do not need to update the request file for every user and email value you want to use.
stepzen request
has two ways to add request variables:
- list them one by one with the
--var
flag, or - read all variables at once from a JSON file with the
--var-file
flag.
For example, with a request file below:
request.graphql
mutation updateUser(
$customer_id: Int!
$email: String!
) {
updateCustomerEmail(
customer_id: $customer_id
email: $email
) {
email
first_name
last_name
}
}
And you can provide variables either inline:
stepzen request "$(cat request.graphql)" \
--var customer_id=5 \
--var email=sam@example.com
Or through a JSON file:
stepzen request "$(cat request.graphql)" --var-file user.json
Which gets the variables from user.json
:
{
"customer_id": 5,
"email": "sam@example.com"
}
Customizing HTTP Headers
The stepzen request
command automatically sets the Content-Type
and Authorization
HTTP headers.
In most cases, this is just enough. However, sometimes you may want to test your GraphQL API with different auth credentials or pass extra HTTP headers if your schema uses the forwardheaders
StepZen feature.
With stepzen request
, you can do that using the -H, --header
flag.
Using a Custom Authorization Header
By default, stepzen request
adds an Authorization: APIKey [your StepZen admin key]
header when calling your GraphQL APIs.
Explicitly adding an Authorization
header with a different value allows you to change that.
For example, you can switch from the admin key to the API key as follows:
stepzen request "$(cat request.graphql)" \
--header "Authorization: APIKey $(stepzen whoami --apikey)"
Another example uses a JWT from the $OPENAI_API_KEY
environment variable instead of the StepZen account keys:
stepzen request "$(cat request.graphql)" \
--header "Authorization: Bearer $OPENAI_API_KEY"
Adding Extra HTTP Headers
When sending requests to a GraphQL API that relies on the forwardheaders
StepZen feature, you can add arbitrary extra HTTP headers. For example,
stepzen request "$(cat request.graphql)" \
-H "User-Agent: curl" \
-H "X-API-Version: 2022-06-01"
Conclusion
stepzen request
is an easy-to-use command line tool for executing GraphQL queries, mutations, and subscriptions.
It simplifies how you interact with GraphQL APIs from a terminal by making it much easier to provide variables, customize headers, and read requests from files.
Currently, stepzen request
is solely for GraphQL APIs deployed to StepZen. It reads the GraphQL API URL from a StepZen workspace configuration and sets the correct authorization headers based on a StepZen account.
Do you want to use stepzen request
with other GraphQL APIs? Visit our Discord Community and let us know.