What GraphQL Can Learn From Databases
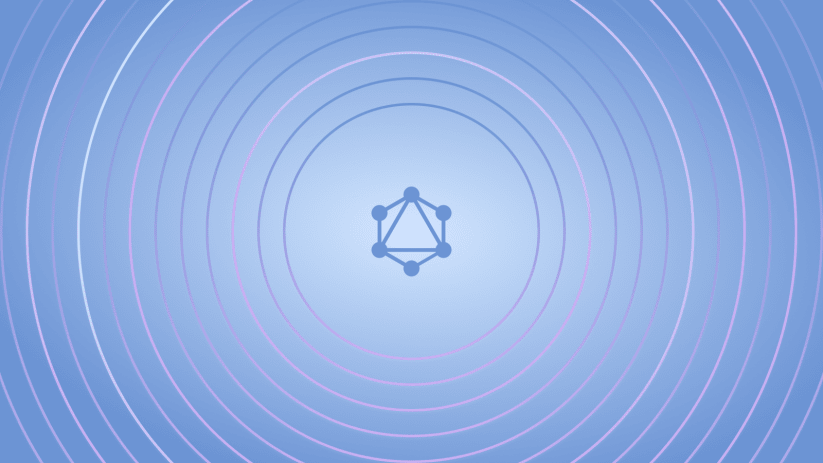
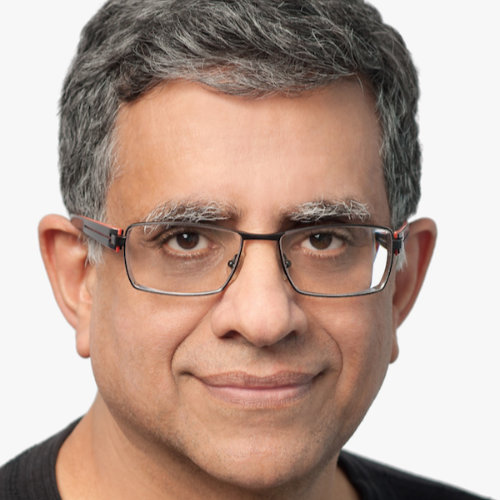
Database systems use declarations (tell me what to do, do not tell me how to do it) to make the overall experience of both the setup and interactive side amazing. In contrast, most GraphQL systems only make the interactive side declarative. The setup side is complex, and worse, the execution of interactions is suboptimal.
It is entirely possible to build a GraphQL system where both sides are declarative, and as a result, the setup is both simple and leads to a much better execution engine.
At StepZen, we believe that GraphQL systems should be set up declaratively.
Databases live in a world of declarative specifications
The database admin (let’s call her Alice) sets up the system using declarative specifications. When she writes “create table x (a int)”, she is telling the database system “what,” and the DBMS then stores the data in its format, creates the right metadata, etc. (the “how”). It is both simple to do (for Alice) and leads to a more intuitive experience and execution for the user who interacts with the database to fetch the data they need.
The end-user (let’s call him Bob) issues interacts through a query specifying “what” he wants (e.g., “select * from x where a = 1”). The database system (DBMS) then converts it into the “how” (for example, understanding that there is an index on (a), that it costs five units to scan x using (a) vs. 20 units to scan linearly through all records, and therefore use index scan). This execution happens easily and optimally because Alice had declared what she wanted.
The two sides — the setup and the interactions — are declarative, and consequently simple to write and code. And they come together to make the execution of the interactions optimal.
Most GraphQL implementations are only half declarative
The interactive side is declarative, so Bob might say:
{
customer (email: "john.doe@example.com") {
name
address }
}
However, typical GraphQL libraries, in any language, be it JavaScript, Java, Go, etc., make the setup side to be programmed. Alice has to write resolvers, which are programmatic snippets describing how each piece of data can be fetched.
There are two problems with this:
-
The program is difficult to write and get right and becomes really difficult to maintain. For example, keys need to be managed. Errors need to be handled. Access control needs to be programmed in. All can be done but at a cost. A cost of skills, time and complexity.
-
Equally bad, because these programmatic snippets are opaque to the GraphQL library, it really messes with the interactive side. For example, there is no knowing how much it costs to fetch a piece of data, and there is no way to rewrite the execution plan. All the magic that databases could do goes out the window. The declarative specification of the query meets the programmatic setup, and bam! Things get clogged.
We really need the setup side to be declarative too
Supposing you declared, during setup, that the resolvers were:
customer: default database lookup
orders (customerId): default database lookup
Customer.orders: call orders (customerId)
How much simpler the code is to write. And maintain. Error conditions are handled by the GraphQL system. Keys are encapsulated in default database lookup
. Access control — can Bob really have access to this information? — is also simplified by factoring the logic into the GraphQL system. The alternative is to pass through the authentication information and leave it to the various backends to deal with authorization.
Rather than passing the authentication information through to the various backends and leaving it to each backend to deal with authorization separately, the logic can be factored into the GraphQL system.
There is a lot of opportunity for optimization. For example, if you ask for:
{
customer (location: "NY") {
address
name
orders {
date
}
}
}
You can issue one query to the database as opposed to hundreds of queries, one for each customer, with a query somewhat like:
select customer.name, customer.address, order.date from customer
join order on (order.customerId = customer.customerId)
where customer.location = "NY"
(The exact syntax is slightly more complicated, but it can be done entirely by the system.)
In case you think that all of this is only when the backend is one database, that is absolutely not true. Such declarative statements:
weather(lat,lon):curl https://openweathermap.org?lat=$lat&lon=$lon
user(name):graphql https://graphql.github.com?user=$user
serve the same purpose. They declare to the GraphQL system “what” has to be done to resolve something. It leads to a simpler, more maintainable GraphQL API for Alice, whose job it is to set up.
But because it is no longer opaque to the GraphQL system, it also leads to better, safer and more optimized interaction for Bob.
You get to eat the cake and have it too.
As you would have guessed, we are firm believers in being declarative on both sides.