Turn Your StepZen GraphQL APIs into REST with Kong
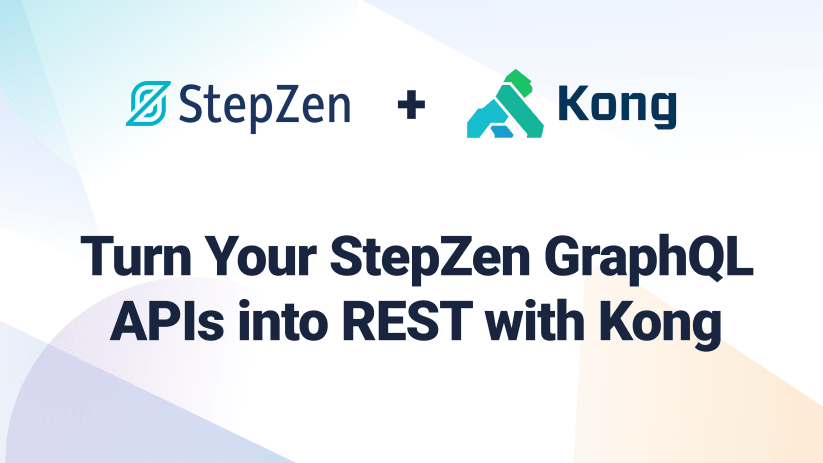
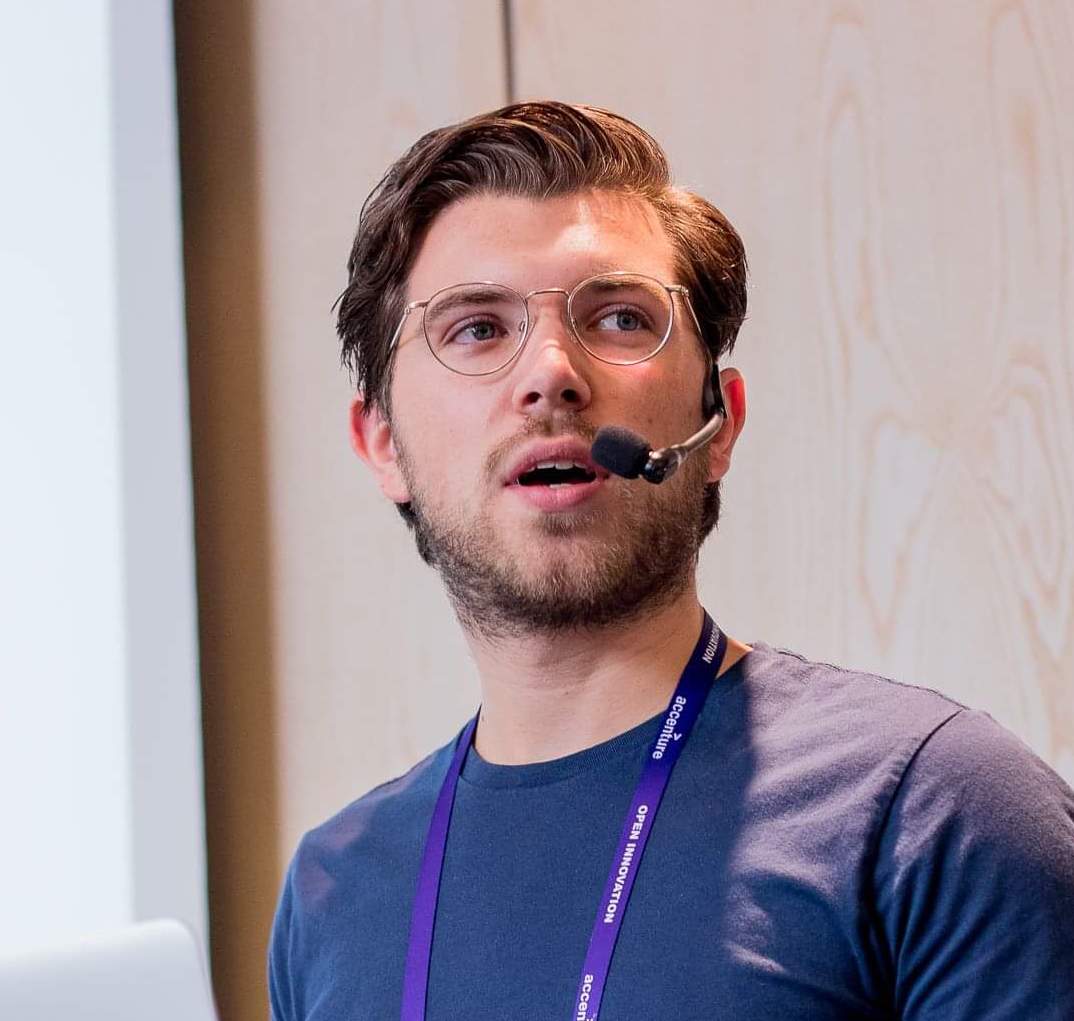
GraphQL APIs are becoming the defacto API standard. But what if you want to use a GraphQL API in a legacy application that only supports REST? This blog post will show you how to use Kong to turn your StepZen GraphQL APIs into REST. Earlier, we published a blog post about how to use Kong as an API Gateway for StepZen GraphQL APIs, including using proxy caching. In this post, we will discuss another reason to use an API Gateway: to turn GraphQL APIs into REST API endpoints.
Why turn GraphQL APIs (back) into REST?
GraphQL is a great way to expose your data to your clients. It allows you to expose only the data your clients need and do so in a way that is easy to use. Although GraphQL might be the most efficient way to consume data in the frontend, it's not the only way to expose your data. REST is still a popular way to expose data, and many systems still rely on it. For example, when you need to integrate your API with a legacy system that only supports REST. Therefore, it might also make sense for your company to expose a REST API.
One way to do so is by calling your GraphQL API the same way as a REST API. Most GraphQL APIs are implemented over HTTP, meaning that the request to a GraphQL API has the same structure as a request to a REST API. The only difference is that every request needs to be a POST
method and the request's body contains a GraphQL query.
Another way is to use an API Gateway to turn your GraphQL API into a REST API. You define the REST API endpoints and map GraphQL queries to these endpoints. This is a more efficient way to expose your GraphQL API as a REST API because you can keep control of the available REST endpoints, and can distribute them to you clients much more like a contract (which REST also is). You can use the API Gateway to expose your GraphQL API as a REST API and to do other things like caching, rate limiting, and authentication.
Using Kong to turn GraphQL APIs into REST APIs
Kong is an open-source API Gateway that you can use with all sorts of APIs, including GraphQL APIs. It helps you to manage your APIs and to do things like caching, rate limiting, and authentication. You can also use it to turn your GraphQL APIs into REST APIs, and we will go over how to do so.
Let's say you have a GraphQL API that converts one currency to another, such as the Frankfurter API in StepZen GraphQL Studio.
# https://graphqldd.stepzen.net/api/dd1cf47f51ac830fe21dc00ec80cee65/__graphql
query ConvertCurrencyByIP ($amount: Float!, $from: String!, $ip: String!) {
converted: ipApi_location(ip: $ip) {
countryCode
price: priceInCountry(amount: $amount, from: $from)
}
}
This query can transform a currency based on the IP address. For example, if you want to convert 100 GBP to USD, you can do so by calling the following query:
curl 'https://graphqldd.stepzen.net/api/dd1cf47f51ac830fe21dc00ec80cee65/__graphql' \
-H 'authority: graphqldd.stepzen.net' \
-H 'accept: application/json' \
-H 'content-type: application/json' \
--data-raw $'{"query":"query ConvertCurrencyByIP($amount: Float\u0021, $from: String\u0021, $ip: String\u0021) { converted: ipApi_location(ip: $ip) { countryCode price: priceInCountry(amount: $amount, from: $from) } }","variables":{"amount":100,"from":"GBP","ip":"8.8.8.8"},"operationName":"ConvertCurrencyByIP"}' \
As StepZen has GraphQL implemented over HTTP, you could already use the call to this GraphQL like a REST API.
However, if you want to use an API Gateway to turn your GraphQL API into a REST API, you can do so by using Kong. In this article we explained how to use Kong as an API Gateway for a StepZen GraphQL API.
The request will look the same when you're using Kong, but the endpoint will be different. Instead of calling the GraphQL endpoint, you will call the Kong endpoint. The Kong endpoint will then call the GraphQL endpoint and return the result to the client, in example if you have a local instance of Kong available on localhost:8000
, you can call the following query:
curl http://localhost:8000/price/ \
--header "Content-Type: application/json" \
--data '{"query": "query ($amount:Float!,$from:String!,$ip:String!){converted:ipApi_location(ip:$ip){countryCode price:priceInCountry(amount:$amount,from:$from)}}", "variables": {"amount":100,"from":"GBP","ip":"8.8.8.8"}}'
{
"data": {
"converted": {
"countryCode": "US",
"price": 115.69
}
}
}
To turn that query into a REST API endpoint, you can use the degraphql plugin that's available for Kong Enterprise. With this plugin, you can create a REST API endpoint and declaratively map the GraphQL query to the REST endpoint. After configuring the plugin, you can call the following endpoint:
curl -X POST http://localhost:8001/services/graphql-demo/degraphql/routes \
--data uri="/gbp/:ip/:amount" \
--data query='query($amount:Float!,$ip:String!){converted:ipApi_location(ip:$ip){countryCode price:priceInCountry(amount:$amount,from:"GBP")}}'
The uri
parameter is used to create the REST endpoint you want to expose, where the :ip
and :amount
parameters are mapped to the parameters in the GraphQL query. The query
parameter is the GraphQL query you want to call.
After registering the new REST endpoint to the plugin, you can call the following endpoint:
curl localhost:8000/price/gbp/8.8.8.8/100
{
"data": {
"converted": {
"countryCode": "US",
"price": 115.69
}
}
}
However, the response has the same structure as earlier for our GraphQL query. This structure is typical for GraphQL APIs but not REST APIs and provides no benefits over calling the GraphQL API directly. To change this, you can "unwrap" the response by using the jq plugin from Kong. You can configure this plugin to remove the data
key from the response and return the data directly.
curl localhost:8001/services/graphql-demo/plugins -d name=jq -d config.response_jq_program='.data' -d config.request_if_media_type='application/json; charset=utf-8'
Now, when you call the REST endpoint, you will get the following response:
curl localhost:8000/price/gbp/8.8.8.8/100
{"converted":{"countryCode":"US","price":115.69}}
This is a much better response for a REST API; you can use this to expose your GraphQL API as a REST API. For a more detailed explanation of how to use Kong to turn GraphQL APIs into REST APIs, you can read this article on the Kong website.
Conclusion
This post shows how you can use Kong to turn your GraphQL APIs into REST APIs. Even though GraphQL is becoming the standard for any client-facing API, many legacy systems still use REST APIs. Using Kong as an API Gateway got GraphQL, you can expose your GraphQL API as a REST API, which these legacy systems can use. This is done by mapping a GraphQL query to a REST endpoint and then using the Kong plugins to transform the response to a REST API response.
Follow us on Twitter or join our Discord community to stay updated about our latest developments.