Analyze Sentiment of Your Blog Comments with StepZen GraphQL Studio
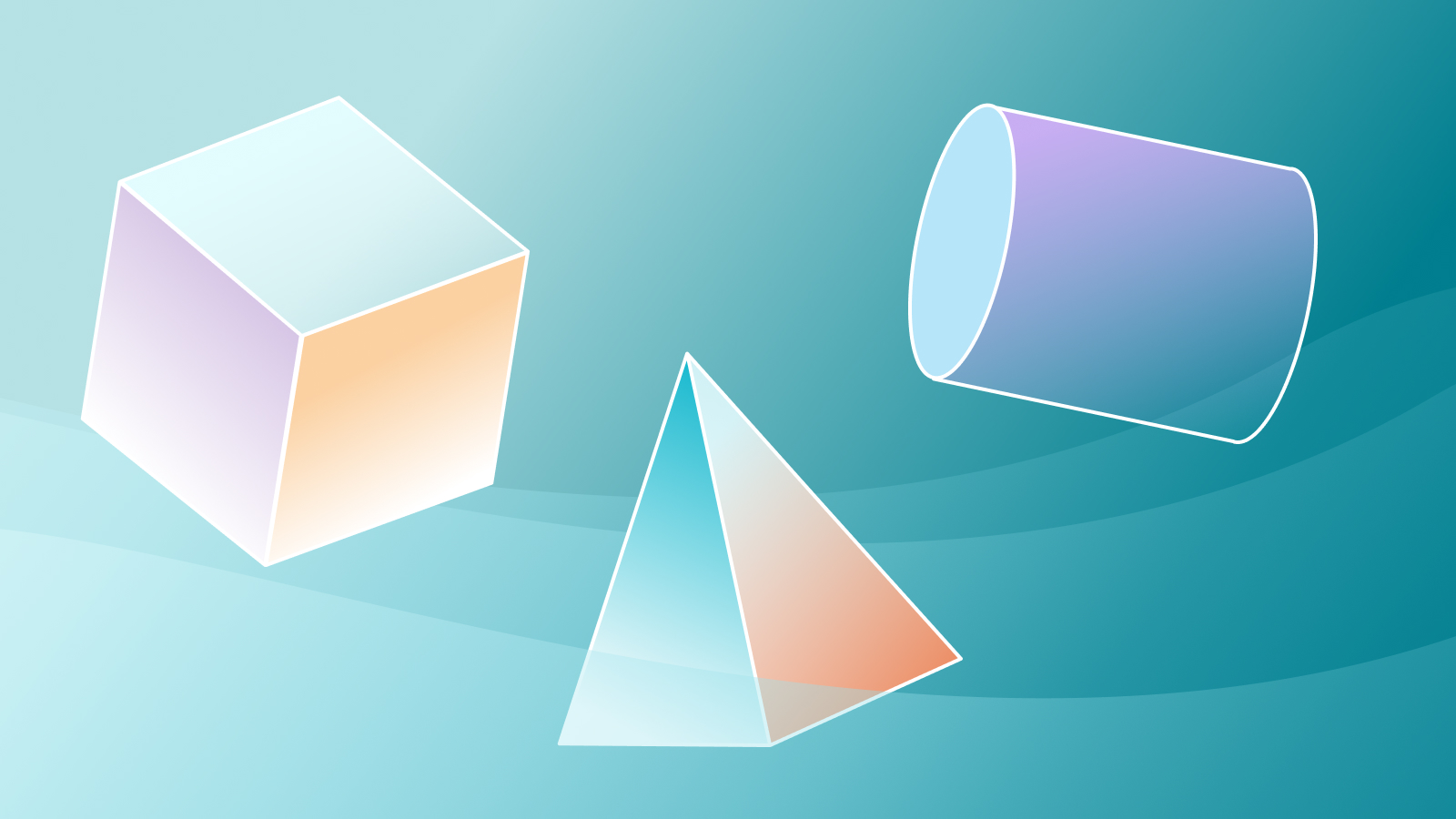
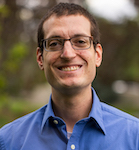
The StepZen GraphQL Studio lets you create your own GraphQL APIs in just a few clicks using a world-class set of professional grade consumer APIs. You do not need to write any resolvers, or even create your own GraphQL schema. Everything is provided for the developer - you can even test queries against mock data. Then all you have to do is include your API keys (which are not stored by StepZen), and start writing queries against your own data.
After constructing your own custom API with these different data sources, you can seamlessly deploy that API to your own custom endpoint. This endpoint could then be accessed by other APIs, or a frontend application, enabling you to build an entire fullstack application.
What are we building?
In this example, we use Google's Cloud Natural Language API and the Dev.to API to analyze the sentiment of blog comments. We can find out whether the comments on your spicy 🥵 hot takes are positive 😊 or negative 😭.
No prerequiste to sign up for an account - or install anything! All we do is visit StepZen GraphQL Studio, select the APIs we want, and go! So let's do it.
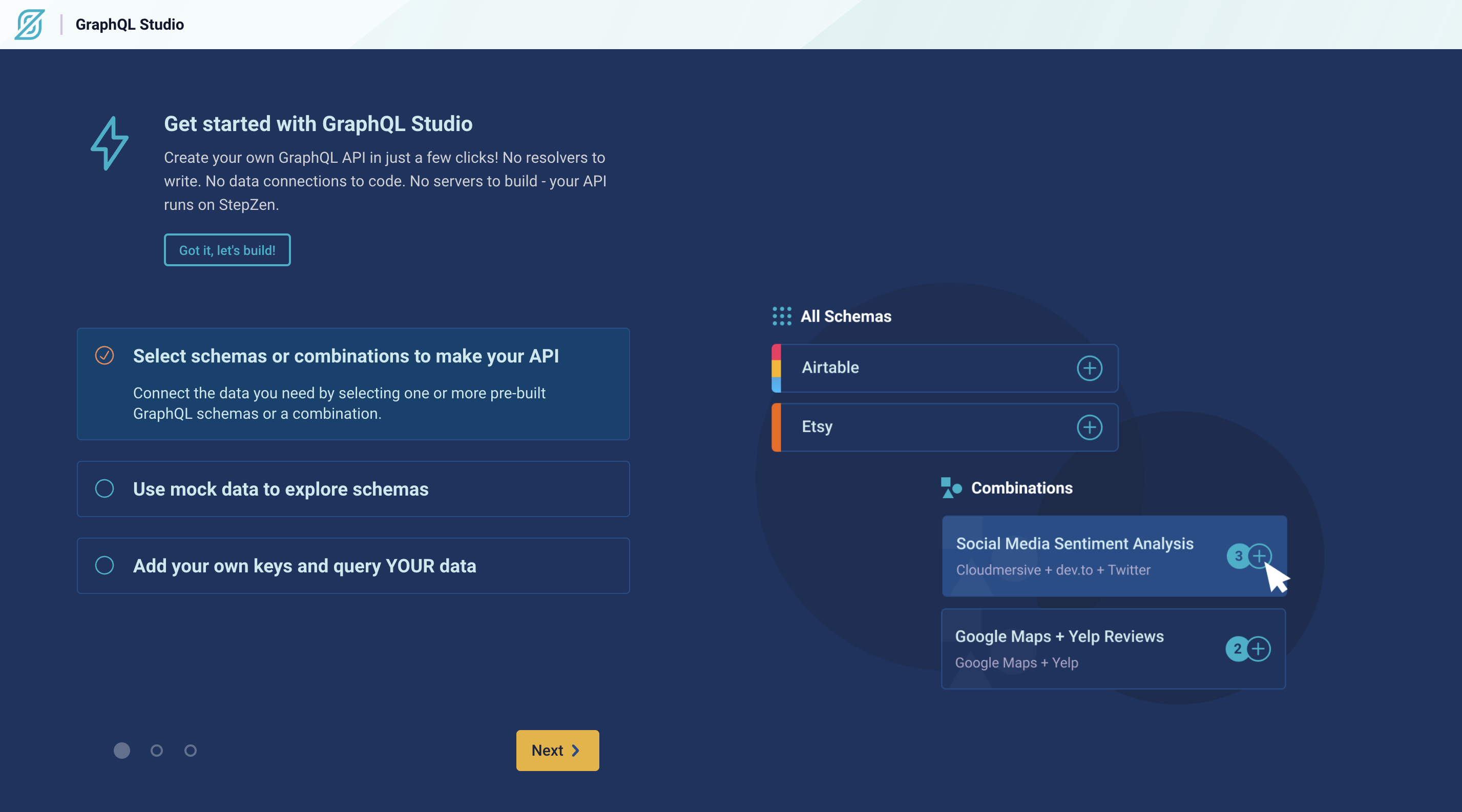
Configure Google Natural Language API
The Cloud Natural Language API from Google provides natural language understanding technologies, such as sentiment analysis, entity recognition, and other text annotations, to developers. In this example we will be utilizing sentiment analysis to assign a numerical score to the positivity or negativity of a given section of text. We will use this capability to assign sentiment analysis scores to the comments on a given Dev.to blog article.
Choose "All Schemas" and scroll down to the letter "G".
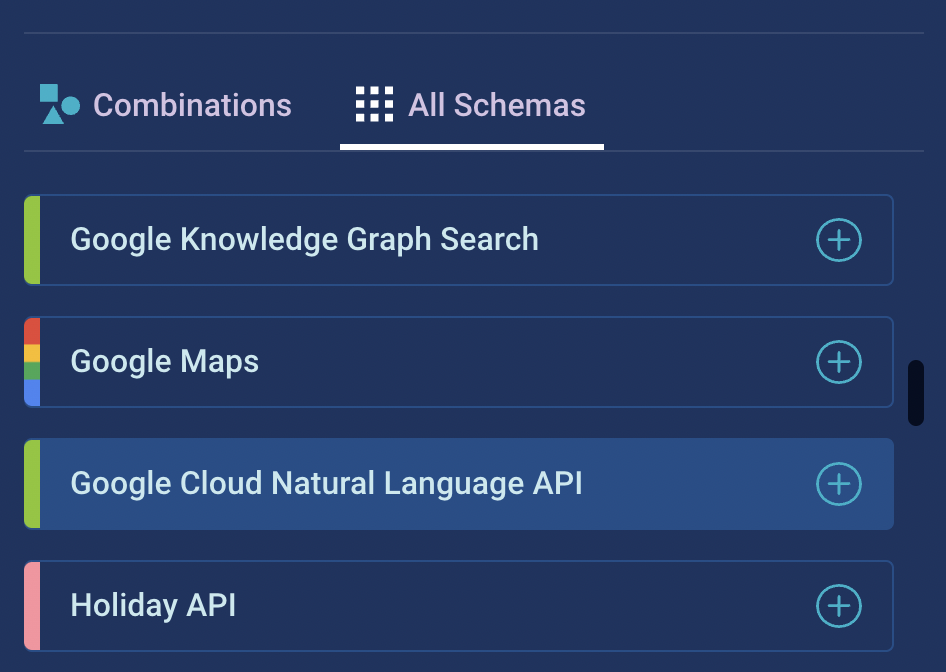
Select "Google Cloud Natural Language API."
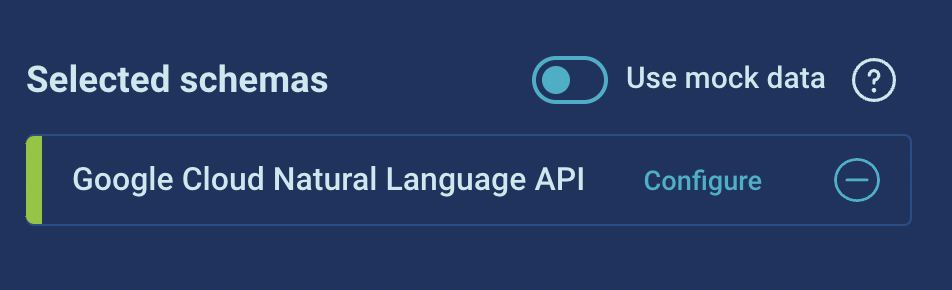
Click "Configure."
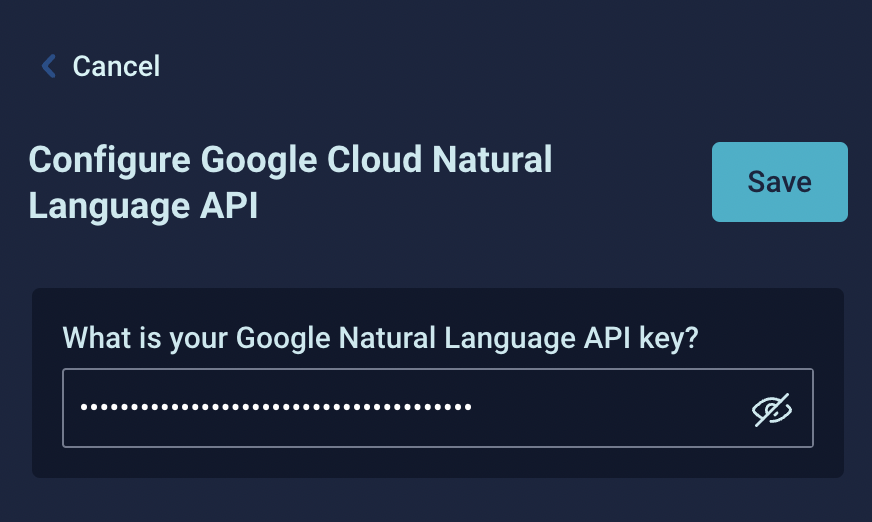
Enter your API key and click "Save."
Run googleNaturalLanguage_analyzeSentiment query
How accurate is Google Natural Language's sentiment analysis? To test it out, we can provide a few pieces of content and see how well the score matches our own common sense interpretation. First we can try the phrase: "I feel happy:"
query GoogleNaturalLanguageQueries(
$googlenaturallanguage_key: Secret!
) {
googleNaturalLanguage_analyzeSentiment(
content: "I feel happy"
googlenaturallanguage_key: $googlenaturallanguage_key
) {
documentSentiment {
score
}
}
}
Output:
{
"data": {
"googleNaturalLanguage_analyzeSentiment": {
"documentSentiment": {
"score": 0.9
}
}
}
}
0.9
means that the response is positive, and also that it is strongly positive, veering close to complete positivity! What if we input, "I feel sad?"
query GoogleNaturalLanguageQueries(
$googlenaturallanguage_key: Secret!
) {
googleNaturalLanguage_analyzeSentiment(
content: "I feel sad"
googlenaturallanguage_key: $googlenaturallanguage_key
) {
documentSentiment {
score
}
}
}
Output:
{
"data": {
"googleNaturalLanguage_analyzeSentiment": {
"documentSentiment": {
"score": -0.9
}
}
}
}
The response is similar to the previous query, but the polar opposite with -0.9
. This means that the response is strongly negative. So what would it mean to get a response right down the middle? How about, "I feel exactly in the middle:"
{
"data": {
"googleNaturalLanguage_analyzeSentiment": {
"documentSentiment": {
"score": 0.5
}
}
}
}
GraphQL Types
How is this being achieved? We can take a look at the schema provided by StepZen:
type GoogleNaturalLanguage_Sentiment @mock {
magnitude: Float
score: Float
}
type GoogleNaturalLanguage_DocumentSentiment @mock {
documentSentiment: GoogleNaturalLanguage_Sentiment
language: String
sentences: [GoogleNaturalLanguage_Sentence]
}
We have a GoogleNaturalLanguage_DocumentSentiment
type which contains the field documentSentiment
that returns GoogleNaturalLanguage_Sentiment
. The GoogleNaturalLanguage_Sentiment
type returns two numbers representing the sentiment score
and magnitude
.
type Query {
googleNaturalLanguage_analyzeSentiment(
content: String!
): GoogleNaturalLanguage_DocumentSentiment
@rest(
endpoint: "https://language.googleapis.com/v1beta2/documents:analyzeSentiment?key=$googlenaturallanguage_key"
method: POST
postbody: """
{"document":{type:"PLAIN_TEXT", "content":"{{ .Get "content" }}"}, "encodingType":"UTF8"}
"""
)
}
The query googleNaturalLanguage_analyzeSentiment
takes a piece of content
that is a String
. The @rest
connector does all the heavy lifting of connecting to the correct endpoint, including your Google API key, and executing the correct POST
request to send the content
.
Dev.to
Dev.to is an open source blogging platform for developers. Dev.to provides a REST API for programmatically accessing and analyzing your blog content. This also includes blog comments. We will perform a query to return an HTML fragment with the contents of a given blog article's comments.
Configure Dev.to Credentials
Return to "All Schemas" and scroll down to the letter "D".
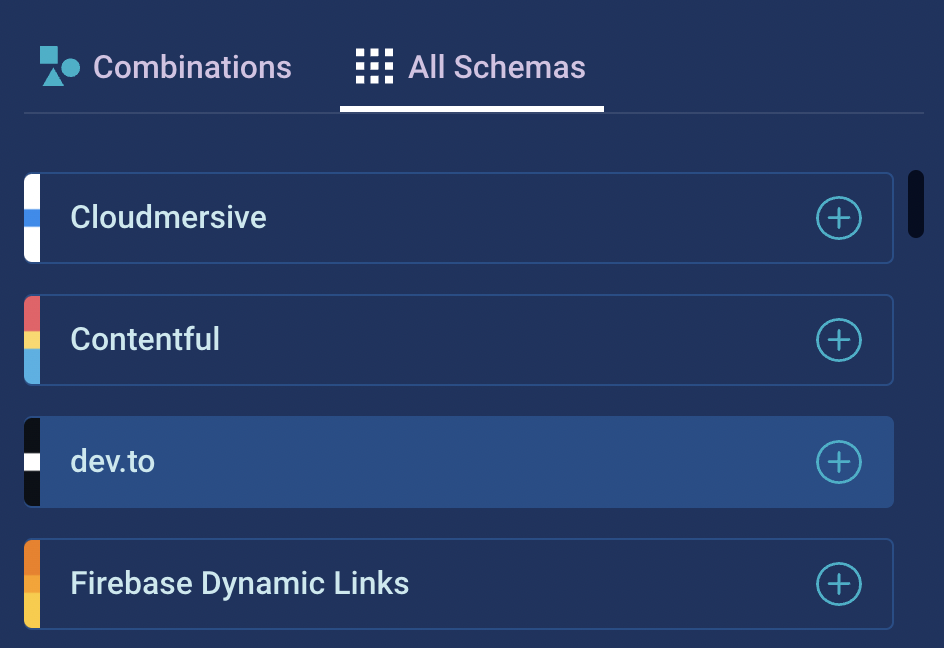
Select "dev.to."
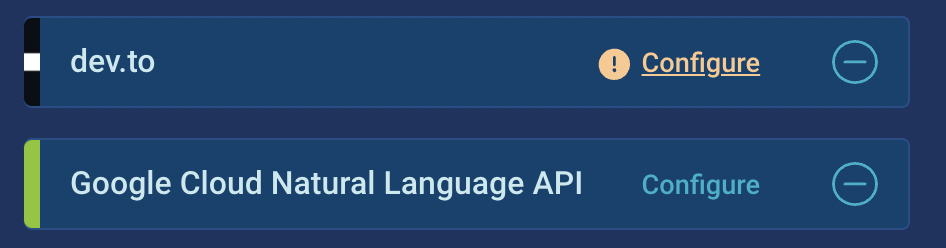
Click "Configure" next to dev.to.
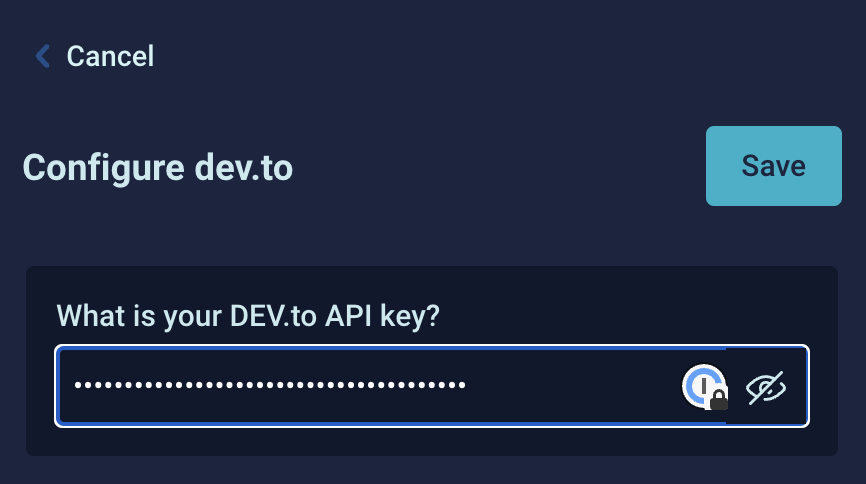
Enter your API key and click "Save."
Run devto_getCommentsByArticleId query
We only need to provide the articles id
, in this case 695280
, which returns the comments for I made 100 CSS loaders for your next project. This particular article was picked because it has over 1,000+ ❤️'s and 2,000+ bookmarks, making it a good sample case for analyzing if the positive sentiment of the comments is picked up by the language API.
query GET_COMMENTS {
devto_getCommentsByArticleId(a_id: 695280) {
body_html
}
}
GraphQL Types
The Devto_getCommentsByArticleIdResponse
type contains a field body_html
of type String
containing the articles comments. It also has a field called children
that returns an array of Devto_DevToComment
types because any comment can have a comment attached to it. This means comments can become deeply nested within other comments.
type Devto_DevToComment {
body_html: String!
children: [Devto_DevToComment!]
created_at: String!
id_code: String!
type_of: String!
}
type Devto_getCommentsByArticleIdResponse {
body_html: String!
children: [Devto_DevToComment!]
created_at: String!
id_code: String!
type_of: String!
}
Our devto_getCommentsByArticleId
query takes in the articles ID (a_id
) and returns a Devto_getCommentsByArticleIdResponse
type. Again we are using the @rest
connector to connect to Dev's REST API for the comments.
type Query {
devto_getCommentsByArticleId(
a_id: Int
): Devto_getCommentsByArticleIdResponse
@rest(endpoint: "https://dev.to/api/comments")
}
Sequence queries with @sequence
Now we can stitch these two queries together by using StepZen's @sequence
directive. The @sequence
directive will chain both queries together and feed the response of the first query into the parameters of the second query. We provide the appropriate steps
and define each query
, first devto_getCommentsByArticleId
and then googleNaturalLanguage_analyzeSentiment
.
type Query {
getCommentSentiment(
a_id: Int
): GoogleNaturalLanguage_DocumentSentiment
@sequence(
steps: [
{
query: "devto_getCommentsByArticleId"
}
{
query: "googleNaturalLanguage_analyzeSentiment",
arguments: [
{ name: "content", field: "body_html" }
]
}
]
)
}
googleNaturalLanguage_analyzeSentiment
takes the arguments
, in this case body_html
, which is transformed into the correct field, content
.
Run getCommentSentiment query
Now we can provide the article ID through a_id
, and return the score
of the articles comments. Lets provide first a score for a popular, well received article:
query GET_COMMENT_SENTIMENT {
getCommentSentiment(a_id: 695280) {
documentSentiment {
score
}
}
}
Output:
{
"data": {
"getCommentSentiment": {
"documentSentiment": {
"score": 0.8
}
}
}
}
If you have a blog on Dev, you might be curious to analyze the sentiment on your own articles. I think I'll try out the comments on my article, Why Am I Hung Up on the Term Fullstack?.
query GET_COMMENT_SENTIMENT {
getCommentSentiment(a_id: 852731) {
documentSentiment {
score
}
}
}
Output:
{
"data": {
"getCommentSentiment": {
"documentSentiment": {
"score": -0.4
}
}
}
}
The output is a -0.4
, representing moderate negativity. Apparently my hot take was just a little bit too spicy. 🥵 🥵
Conclusion
This combination represents only one of hundreds of possible combinations of APIs available through the StepZen GraphQL Studio. None of the code contained in this article would need to be written by a new user of these APIs, it is already provided at no cost to the developer. GraphQL's built-in documentation and schema introspection allows a developer to quickly begin writing queries against this wide array of APIs.
To try out the GraphQL Studio yourself, visit graphql.stepzen.com. We'd love to know what you build - see you over on Discord.